Introduction
Welcome to the Backer API.
Overview
This document describes how to integrate with Backer using the Backer REST API.
Here are the flows to integrate in:
- Register a new user
- Create and establish a new fund
- Contribute to the fund
Flow To Register A New User
This is the flow to register a new user.
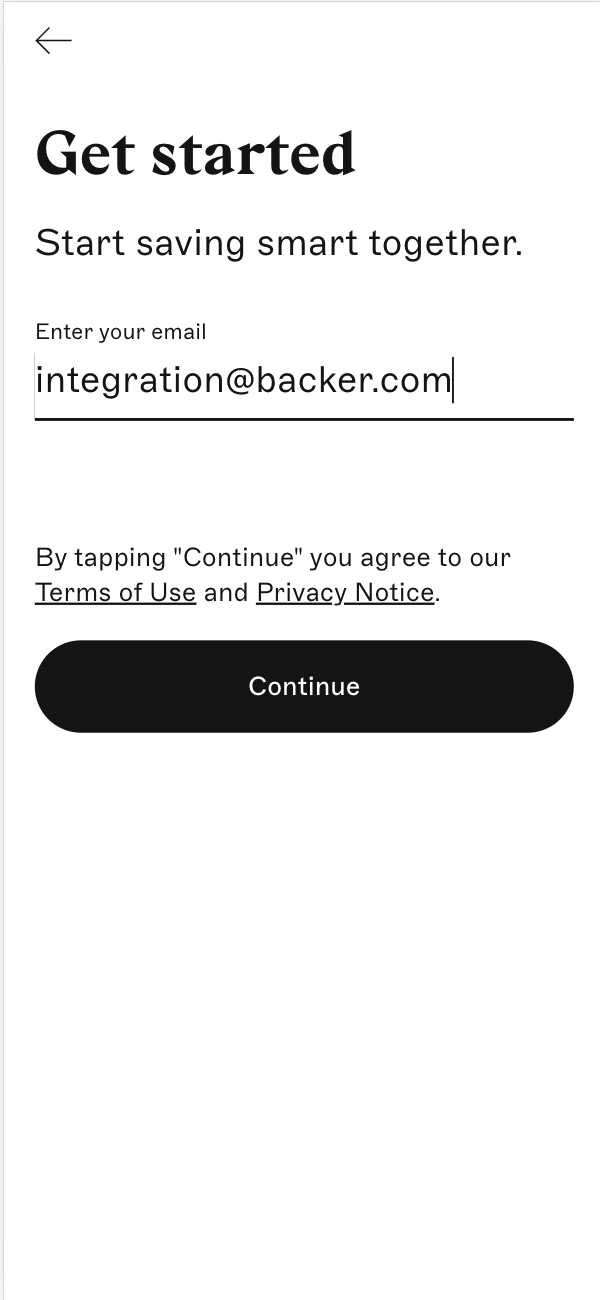
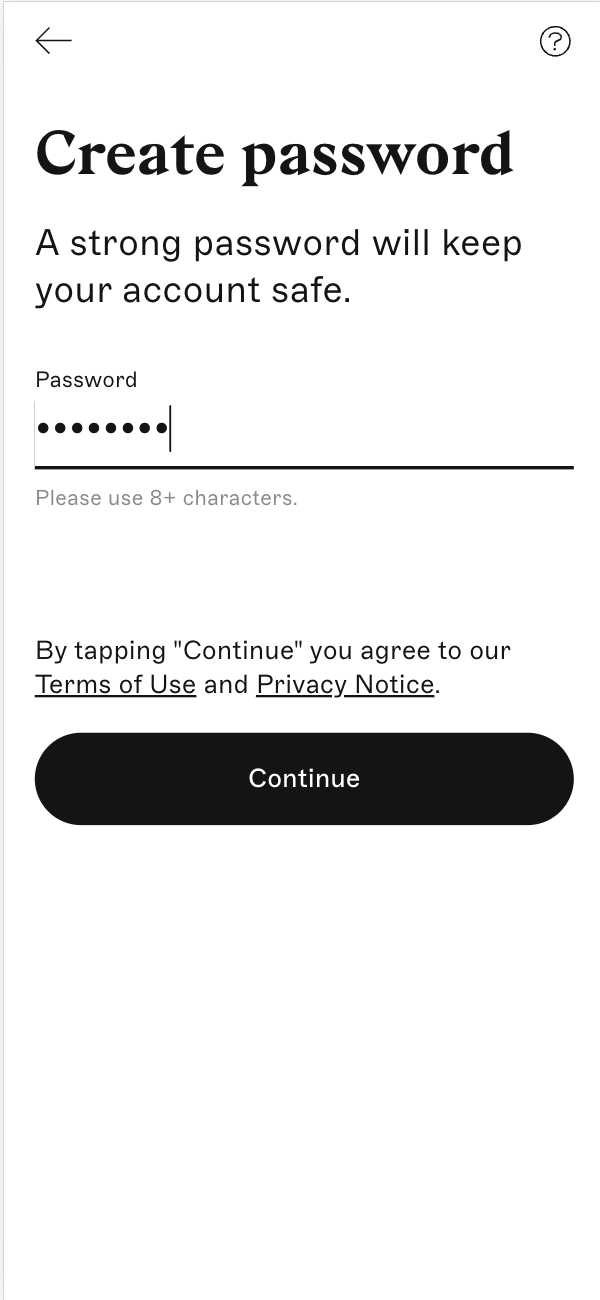
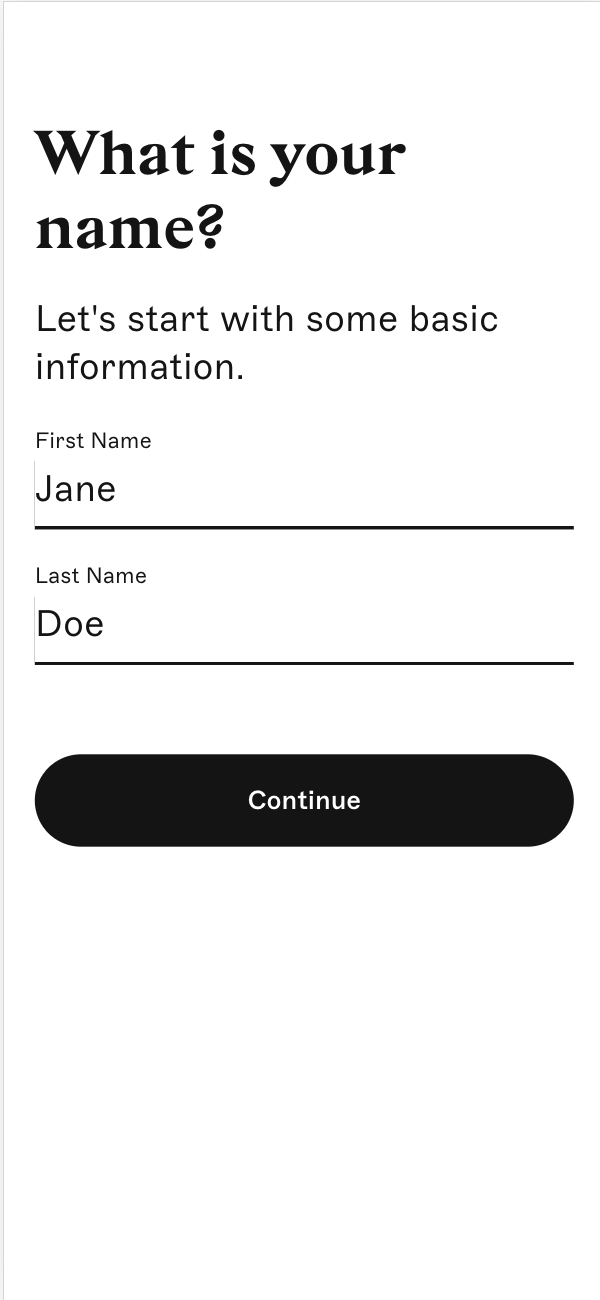
Flow To Establish A New Fund
This is the flow to provision a fund and establish it. You need to specify the user and fund beneficiary information necessary to open the 529 account, the fund name and the risk level chosen by the user (used by Backer to select a portfolio). Once this is done there is a last step for the user to answer a few identity related questions on the 529 plan provider website so their 529 account can be successfully opened.
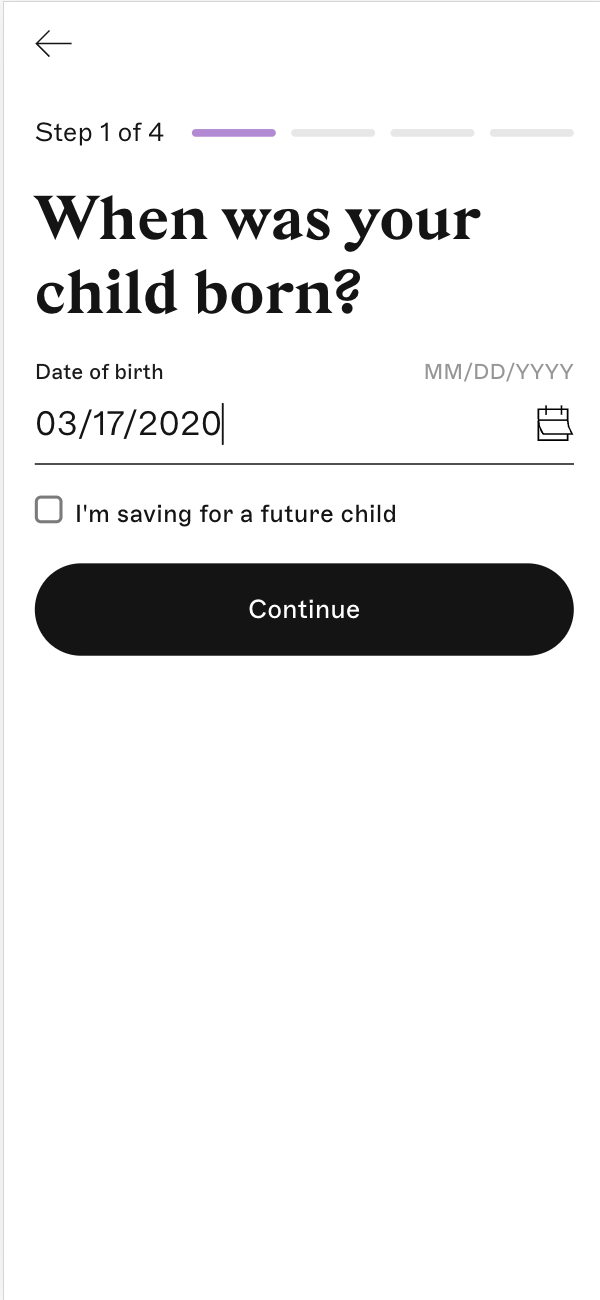
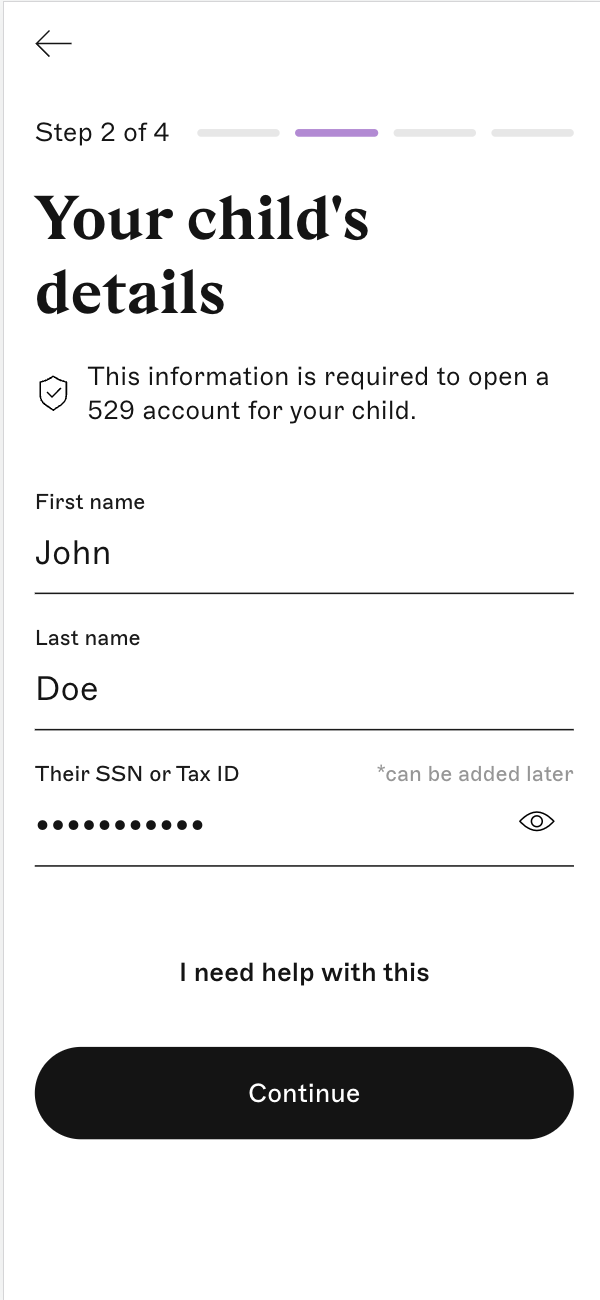
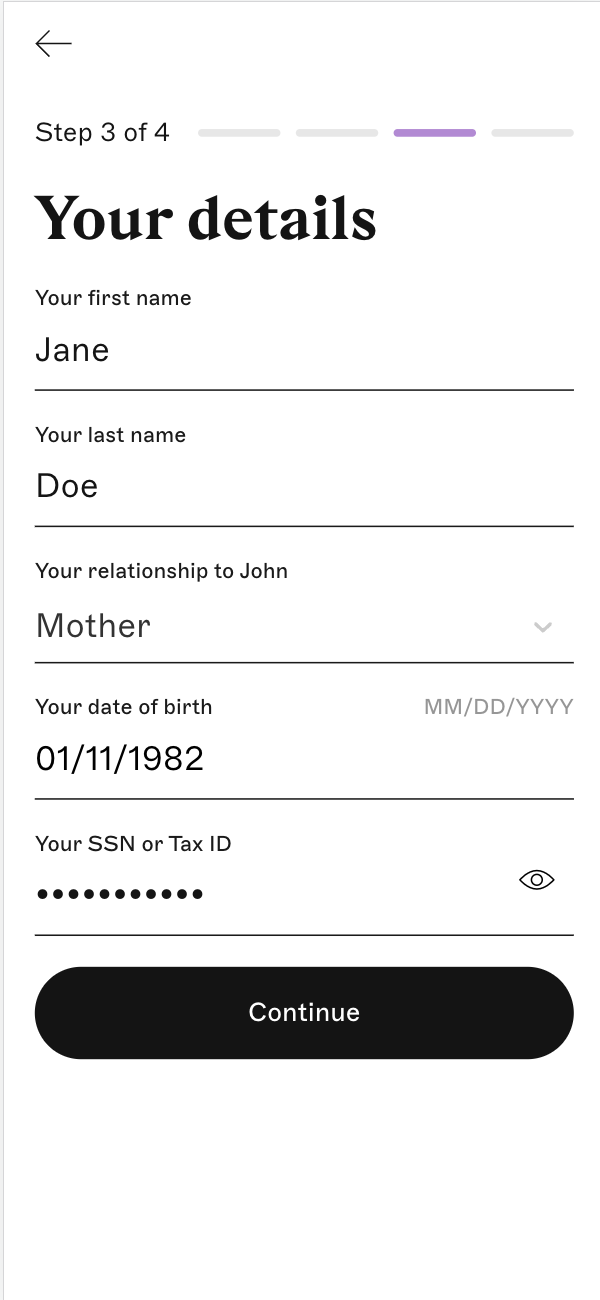
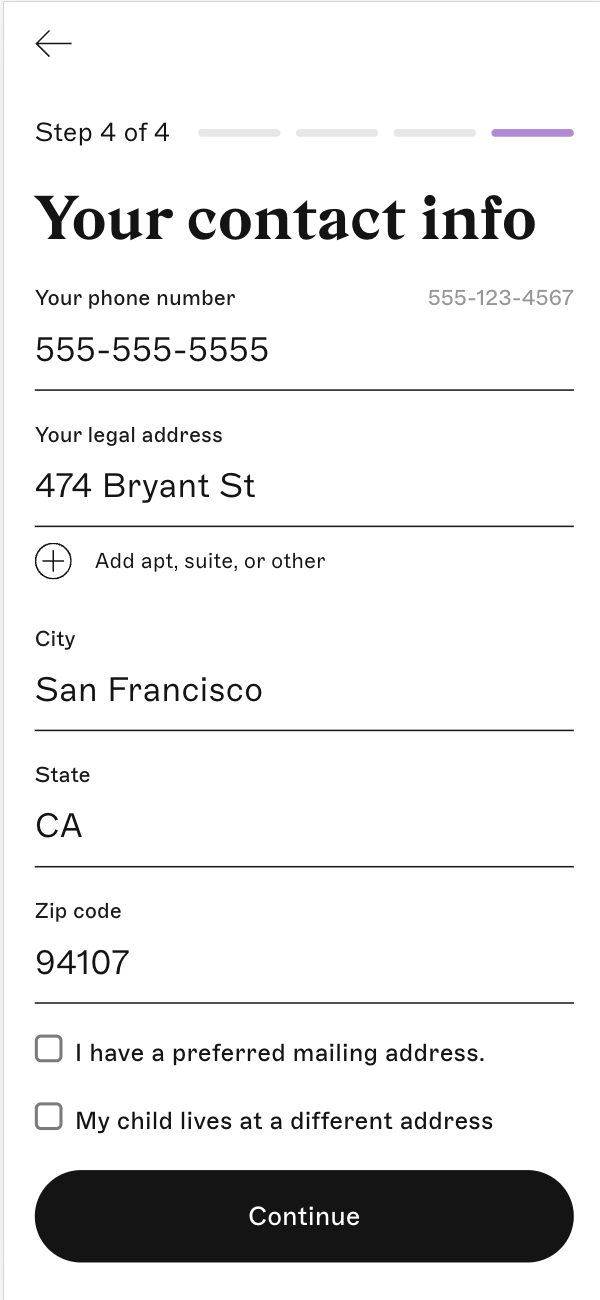
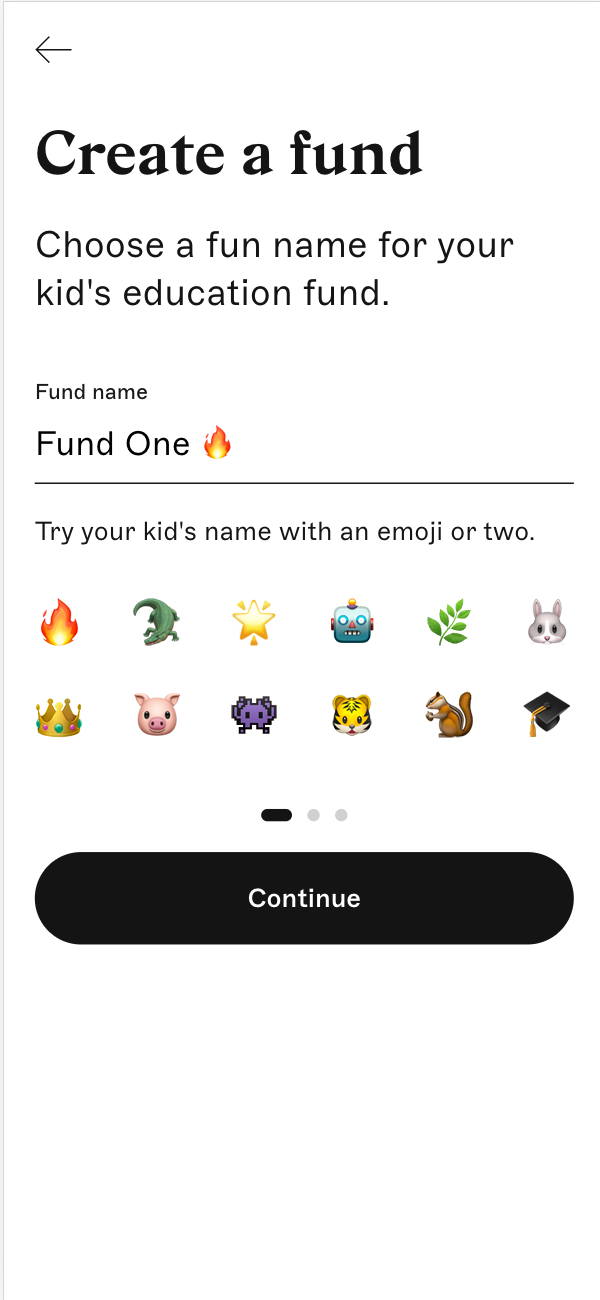
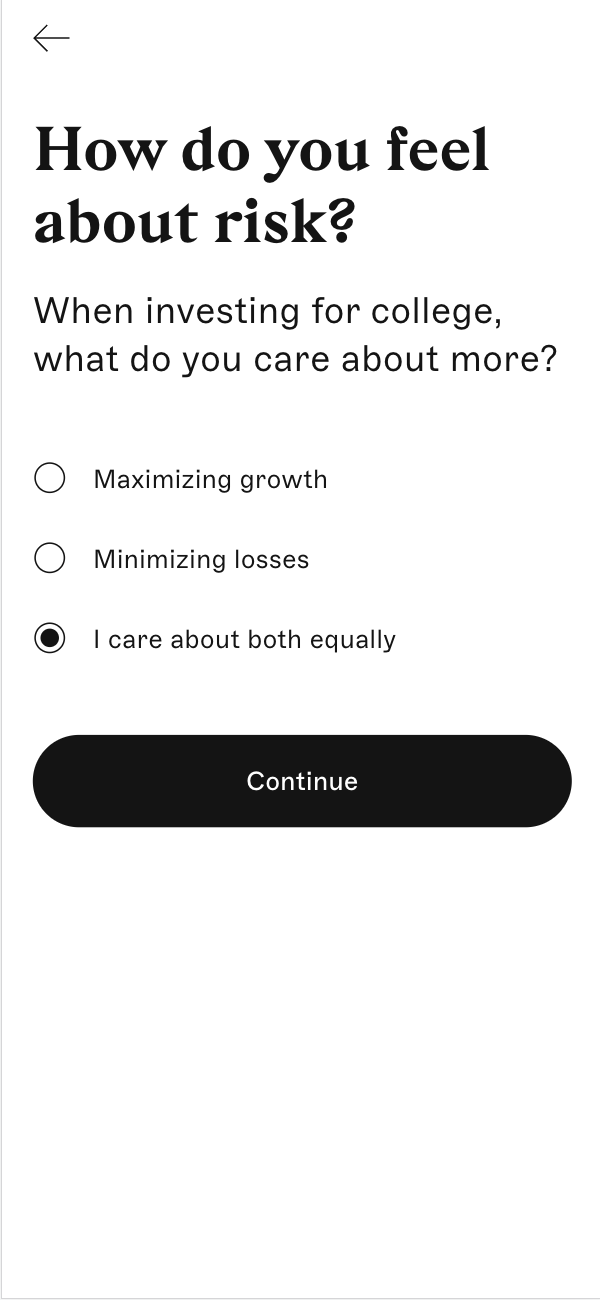
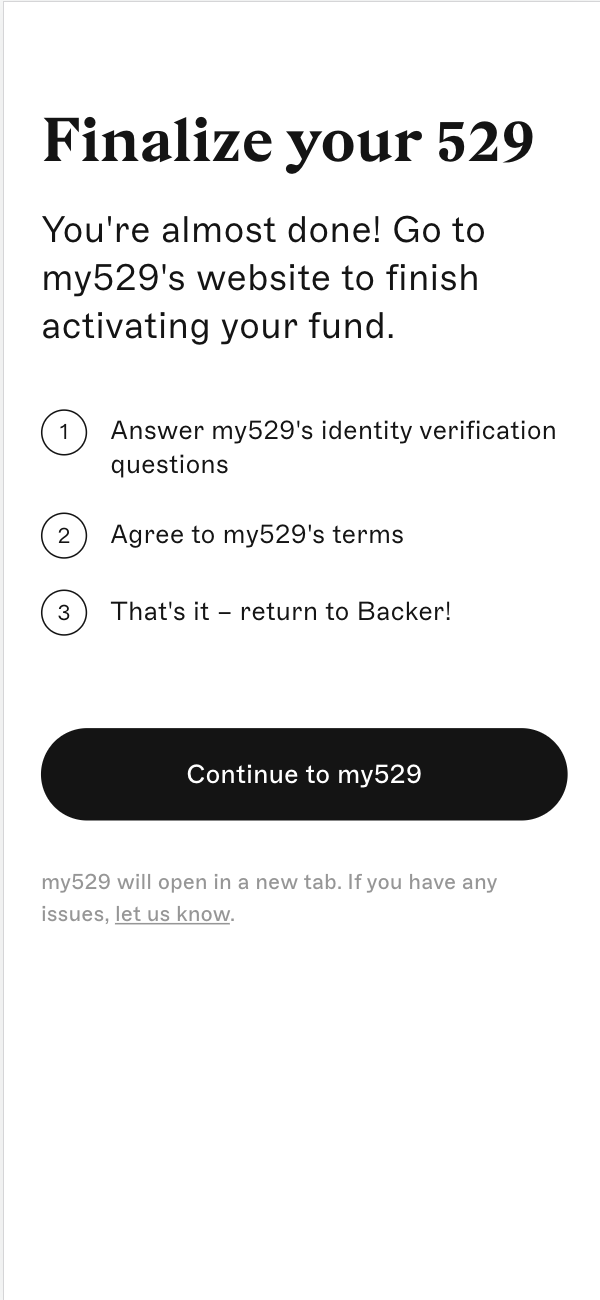
Flow To Contribute To The Fund
There are two ways to integrate with fund contributions:
- have Backer schedule payments from the contributions based on their frequency or
- have the partner schedule the payments themselves
In both cases the Backer application will pull the funds from the partner bank account using ACH transfers.
When the user decides to contribute to the fund with a one time or recurring payments, they create a contribution by specifying frequency and amount.
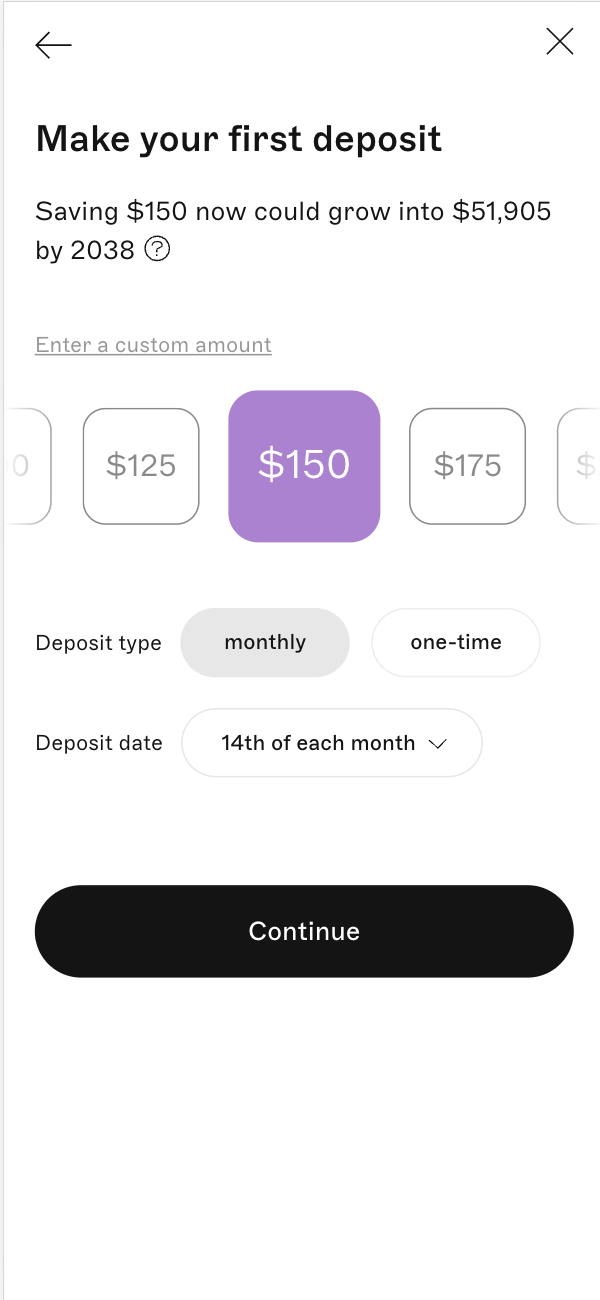
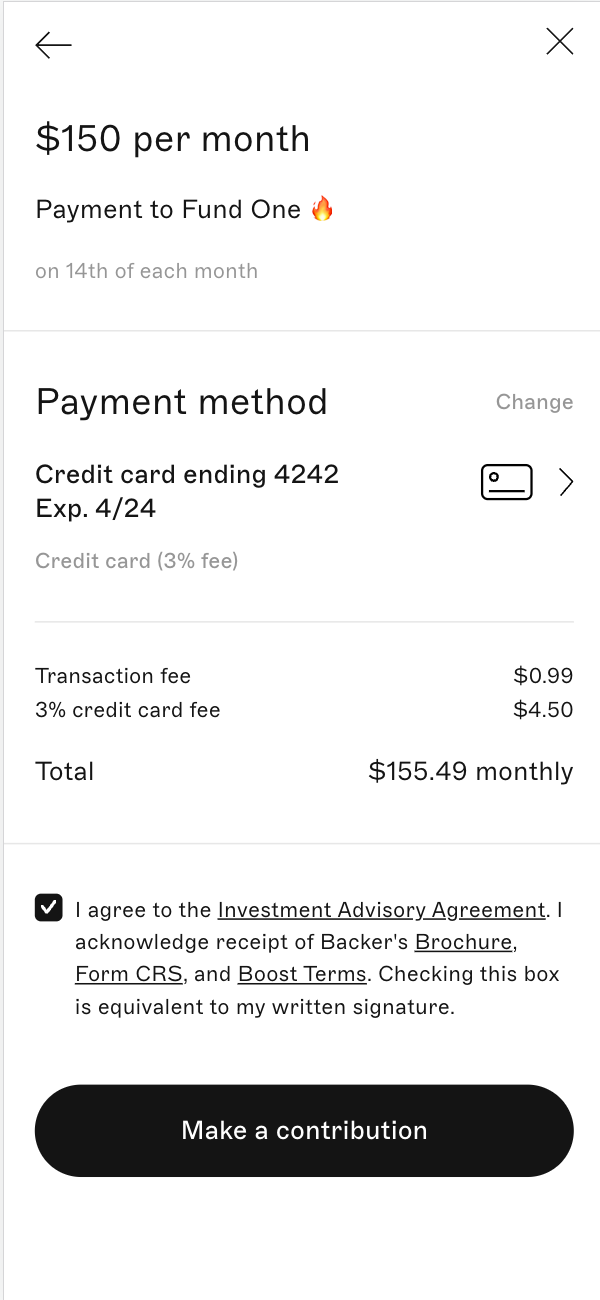
Authentication
In order to use the Backer API, you must provide your API token for each request.
The Backer team will provide you with a unique API token for your account.
To authorize, use this code:
# You can just pass the correct header with each request
curl "https://backer.com/restapi/v1/api-endpoint-here" \
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: <API token>"
Make sure to replace
<API token>
with your own API token.
Backer uses API keys to allow access to the API.
Backer expects for the API token to be included in all API requests to the server in a header that looks like the following:
Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c
Users
Get User By External ID
curl "https://backer.com/restapi/v1/users/search/USR123-456" \
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
The above command returns JSON structured like this:
{
"uuid": "6a62fadd-6f95-4549-9d3f-b625716ccbbd",
"external_id": "USR123-456",
"email": "user@example.com",
"inserted_at": "2019-10-08T17:39:18.179490Z",
"first_name": "Jane",
"last_name": "Doe",
"middle_name": "Arianne",
"photo_url": "https://res.cloudinary.com/myphoto.png"
}
This endpoint returns an existing user.
HTTP Request
GET https://backer.com/restapi/v1/users/search/<external_id>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
external_id | string | yes | External user ID used to register this user. |
Get User
curl "https://backer.com/restapi/v1/users/6a62fadd-6f95-4549-9d3f-b625716ccbbd" \
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
The above command returns JSON structured like this:
{
"uuid": "6a62fadd-6f95-4549-9d3f-b625716ccbbd",
"email": "user@example.com",
"external_id": "USR123-456",
"inserted_at": "2019-10-08T17:39:18.179490Z",
"first_name": "Jane",
"last_name": "Doe",
"middle_name": "Arianne",
"photo_url": "https://res.cloudinary.com/myphoto.png"
}
This endpoint returns the requested user.
HTTP Request
GET https://backer.com/restapi/v1/users/<uuid>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of the user to fetch. |
Register User
curl "https://backer.com/restapi/v1/users" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"external_id": "USR123-456",
"first_name": "Jane",
"last_name": "Doe",
"middle_name": "Arianne",
"email": "jane@example.com"}'
The above command returns JSON structured like this:
{
"email": "jane@example.com",
"uuid": "e95d1ea1-9f5b-44d6-822f-ad4a481ba2c8",
"external_id": "USR123-456",
"inserted_at": "2019-10-08T17:39:18.179490Z",
"first_name": "Jane",
"last_name": "Doe",
"middle_name": "Arianne"
}
This endpoint registers a new user.
HTTP Request
POST https://backer.com/restapi/v1/users
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
external_id | string | yes | ID of the user in your own application. |
first_name | string | yes | User first name |
last_name | string | yes | User last name |
middle_name | string | no | User middle name |
string | yes | User email |
Funds
Establish A New Fund For A Kid
curl "https://backer.com/restapi/v1/users/e95d1ea1-9f5b-44d6-822f-ad4a481ba2c8/funds" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"prebirth": false,
"beneficiary_first_name": "John",
"beneficiary_last_name": "Doe",
"beneficiary_born_on": "2020-03-17",
"beneficiary_ssn": "123456789",
"beneficiary_physical_address_street": "474 Bryant St",
"beneficiary_physical_address_zip": "94107",
"beneficiary_physical_address_city": "San Francisco",
"beneficiary_physical_address_state": "CA",
"owner_first_name": "Jane",
"owner_middle_name": "Arianne",
"owner_last_name": "Doe",
"owner_born_on": "1982-01-11",
"owner_relationship": "mother",
"owner_ssn": "321654987",
"owner_phone": "5555555555",
"owner_physical_address_street": "474 Bryant St",
"owner_physical_address_zip": "94107",
"owner_physical_address_city": "San Francisco",
"owner_physical_address_state": "CA",
"owner_mailing_address_street": "474 Bryant St",
"owner_mailing_address_zip": "94107",
"owner_mailing_address_city": "San Francisco",
"owner_mailing_address_state": "CA",
"risk_level": "maximizing_gains",
"fund_name": "John\'s College Fund"}'
The above command returns JSON structured like this:
{
"uuid": "c516af7e-69f4-4a9f-ad50-32524bd4a0c8",
"establishment_url": "https://login.my529.org/poaaccountapplication?key=423acf31-0399-482a-83f1-5a9aa3e72a18",
"fund_name": "John\'s College Fund",
"handle": "johnscollegefund123"
}
This endpoint requests to establish a new fund. We can establish a fund from the owner and beneficiary data, the risk level selected by the user and a fund name, that is displayed on the public fund page.
HTTP Request
POST https://backer.com/restapi/v1/users/<uuid>/funds
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | User UUID |
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
prebirth | boolean | yes | false (if true account is for a future baby, else it is for a kid) |
beneficiary_first_name | string | yes | kid first name |
beneficiary_middle_name | string | no | kid middle name |
beneficiary_last_name | string | yes | kid last name |
beneficiary_born_on | date (iso8601) | yes | kid birth date |
beneficiary_ssn | string | yes | kid social security number |
beneficiary_physical_address_street | string | yes | kid physical address: street line 1 |
beneficiary_physical_address_street2 | string | no | kid physical address: street line 2 |
beneficiary_physical_address_zip | string | yes | kid physical address: zip code |
beneficiary_physical_address_city | string | yes | kid physical address: city |
beneficiary_physical_address_state | string | yes | kid physical address: state |
owner_first_name | string | yes | user first name |
owner_middle_name | string | no | user middle name |
owner_last_name | string | yes | user last name |
owner_born_on | date | yes | user birth date |
owner_relationship | relationship | yes | user relationship to the kid |
owner_ssn | string | yes | kid social security number |
owner_phone | phone | yes | user phone number |
owner_physical_address_street | string | yes | user physical address: street line 1 |
owner_physical_address_street2 | string | no | user physical address: street line 2 |
owner_physical_address_zip | string | yes | user physical address: zip code |
owner_physical_address_city | string | yes | user physical address: city |
owner_physical_address_state | string | yes | user physical address: state |
owner_mailing_address_street | string | yes | user physical address: street line 1 |
owner_mailing_address_street2 | string | no | user physical address: street line 2 |
owner_mailing_address_zip | string | yes | user physical address: zip code |
owner_mailing_address_city | string | yes | user physical address: city |
owner_mailing_address_state | string | yes | user physical address: state |
risk_level | risk_level | yes | investment risk level |
fund_name | string | yes | name of the fund |
Establish A New Fund For Future Baby
curl "https://backer.com/restapi/v1/users/e95d1ea1-9f5b-44d6-822f-ad4a481ba2c8/funds" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"prebirth": true,
"owner_first_name": "John",
"owner_last_name": "Frusciante",
"owner_born_on": "1970-03-05",
"owner_ssn": "321654987",
"owner_phone": "5555555555",
"owner_physical_address_street": "4900 Marie P DeBartolo Way",
"owner_physical_address_zip": "95054",
"owner_physical_address_city": "Santa Clara",
"owner_physical_address_state": "CA",
"owner_mailing_address_street": "4900 Marie P DeBartolo Way",
"owner_mailing_address_zip": "95054",
"owner_mailing_address_city": "Santa Clara",
"owner_mailing_address_state": "CA",
"risk_level": "maximizing_gains",
"fund_name": "John\'s College Fund"}'
The above command returns JSON structured like this:
{
"uuid": "c516af7e-69f4-4a9f-ad50-32524bd4a0c8",
"establishment_url": "https://login.my529.org/poaaccountapplication?key=423acf31-0399-482a-83f1-5a9aa3e72a18",
"fund_name": "John\'s College Fund",
"handle": "johnscollegefund123"
}
This endpoint requests to establish a new fund. We can establish a fund from the owner and beneficiary data, the risk level selected by the user and a fund name, that is displayed on the public fund page.
HTTP Request
POST https://backer.com/restapi/v1/users/<uuid>/funds
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | User UUID |
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
prebirth | boolean | yes | true (if true account is for a future baby, else it is for a kid) |
owner_first_name | string | yes | user first name |
owner_middle_name | string | no | user middle name |
owner_last_name | string | yes | user last name |
owner_born_on | date | yes | user birth date |
owner_ssn | string | yes | kid social security number |
owner_phone | phone | yes | user phone number |
owner_physical_address_street | string | yes | user physical address: street line 1 |
owner_physical_address_street2 | string | no | user physical address: street line 2 |
owner_physical_address_zip | string | yes | user physical address: zip code |
owner_physical_address_city | string | yes | user physical address: city |
owner_physical_address_state | string | yes | user physical address: state |
owner_mailing_address_street | string | yes | user physical address: street line 1 |
owner_mailing_address_street2 | string | no | user physical address: street line 2 |
owner_mailing_address_zip | string | yes | user physical address: zip code |
owner_mailing_address_city | string | yes | user physical address: city |
owner_mailing_address_state | string | yes | user physical address: state |
risk_level | risk_level | yes | investment risk level |
fund_name | string | yes | name of the fund |
Link An Existing Account To A Fund
curl "https://backer.com/restapi/v1/linked_funds" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{
"plan_id": 3,
"account_number": "1234567890",
"beneficiary_first_name": "John",
"beneficiary_last_name": "Doe",
"fund_name": "John's College Fund",
}'
The above command returns JSON structured like this:
{
"uuid": "3ad7f2a1-5add-4ae7-942b-0690ad35f2c2",
"fund_name": "John\'s College Fund",
"handle": "johnscollegefund987",
"plan_id": 3,
"plan_provider": "BlackRock CollegeAdvantage Advisor 529 Savings Plan",
"account_number": "1234567890"
}
This endpoint requests to link a new fund to an existing 529 plan.
HTTP Request
POST https://backer.com/restapi/v1/linked_funds
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
plan_id | integer | yes | ID of the 529 plan provider to link to |
account_number | string | yes | 529 account number |
beneficiary_first_name | string | yes | first name consistent with 529 account |
beneficiary_last_name | string | yes | last name consistent with 529 account |
fund_name | string | yes | name of the fund |
Get A Fund
curl "https://backer.com/restapi/v1/funds/c516af7e-69f4-4a9f-ad50-32524bd4a0c8" \
-H 'Content-Type: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
The above command returns JSON structured like this:
{
"uuid": "c516af7e-69f4-4a9f-ad50-32524bd4a0c8",
"establishment_url": "https://login.my529.org/poaaccountapplication?key=423acf31-0399-482a-83f1-5a9aa3e72a18",
"fund_name": "John\'s College Fund",
"handle": "johnscollegefund123",
"public_url": "https://backer.com/johncollegefund123",
"photo_url": "https://res.cloudinary.com/johnsphoto.png",
"description": "With your help, we can ensure that John gets to pursue as much education as they want.",
"is_established": true,
"settled_balance": 231867,
"settled_balance": 231867,
"pending_balance": 251867,
"plan_id": 60,
"plan_provider": "my529 - Utah",
"account_number": "901939500",
"portfolio_label": "Target Enrollment 2032/2033",
"established_at": "2018-03-29T08:27:34.365846Z"
}
This endpoint fetches a fund.
HTTP Request
GET https://backer.com/restapi/v1/funds/<uuid>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of the fund to get |
Update A Fund
curl "https://backer.com/restapi/v1/funds/c516af7e-69f4-4a9f-ad50-32524bd4a0c8" \
-X PUT
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"fund_name": "John\'s Fund 🎓", "handle": "johnsfund489"}'
The above command returns JSON structured like this:
{
"uuid": "c516af7e-69f4-4a9f-ad50-32524bd4a0c8",
"establishment_url": "https://login.my529.org/poaaccountapplication?key=423acf31-0399-482a-83f1-5a9aa3e72a18",
"fund_name": "John\'s Fund 🎓",
"handle": "johnsfund489",
"public_url": "https://backer.com/johncollegefund123",
"photo_url": "https://res.cloudinary.com/johnsphoto.png",
"description": "With your help, we can ensure that John gets to pursue as much education as they want.",
"is_established": true,
"settled_balance": 231867,
"pending_balance": 251867,
"plan_provider": "my529 - Utah",
"account_number": "901939500",
"portfolio_label": "Target Enrollment 2032/2033",
"established_at": "2018-03-29T08:27:34.365846Z"
}
This endpoint updates fund data.
HTTP Request
PUT https://backer.com/restapi/v1/funds/<uuid>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of the fund to get |
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
fund_name | string | no | name of the fund |
handle | string | no | unique handle of the fund for the public fund URL |
photo_url | URL | no | photo of the fund beneficiary |
description | string | no | description of the fund displayed on publid fund page |
Initiate A Withdrawal
curl "https://backer.com/restapi/v1/funds/c516af7e-69f4-4a9f-ad50-32524bd4a0c8/initiate_withdrawal" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"amount": 14595, "expense_type": "K-12"}'
The above command returns JSON structured like this:
{
"uuid": "c516af7e-69f4-4a9f-ad50-32524bd4a0c8",
"amount": 14595,
"expense_type": "K-12"
}
This endpoint requests to to withdraw funds from the user 529 account.
HTTP Request
POST https://backer.com/restapi/v1/funds/<uuid>/initiate_withdrawal
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of the fund to initiate a withdrawal on |
amount | cents | yes | amount in cents |
expense_type | expense_type | yes | Type of expense |
Fetch Transactions
curl "https://backer.com/restapi/v1/funds/c516af7e-69f4-4a9f-ad50-32524bd4a0c8/transactions" \
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
The above command returns JSON structured like this:
[
{
"uuid": "ff50d90a-0870-460c-9ad7-83629737a411",
"amount": 10000,
"description": "Contribution from Jane Arianne Doe",
"occurred_on": "2022-09-21",
"status": "Settled",
"type": "contribution",
"frequency": "monthly"
},
{
"uuid": "71fffd07-c203-43c5-b69e-c3ac30259b3f",
"amount": 25000,
"description": "Gift from Karin McFarlane",
"occurred_on": "2022-08-18",
"status": "Settled",
"type": "gift",
"frequency": "onetime"
}
]
This endpoint returns the transactions for a fund.
HTTP Request
GET https://backer.com/restapi/v1/funds/<uuid>/transactions
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of the fund to fetch the transactions from |
Contributions
Users send funds to their 529 account(s) by making contributions. A contribution can be a one-time payment or it can be set up as a recurring payment: monthly, twice a month, or annually.
Specific mechanisms for transferring funds between the partner and Backer will depend on the partner's payment infrastructure and is out side of the scope of this document.
Funds are transferred to the user's 529 account by Backer.
Create Contribution
curl "https://backer.com/restapi/v1/funds/c516af7e-69f4-4a9f-ad50-32524bd4a0c8/contributions" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{
"frequency": "monthly",
"amount": 15000,
"first_day": 14
}'
The above command returns JSON structured like this:
{
"uuid": "56bb5278-1df8-46b2-93a0-dfc312bb54a4",
"frequency": "monthly",
"amount": 15000,
"status": "scheduled",
"activation_date": "2022-10-14",
"first_day": 14,
"second_day": null
}
This endpoint creates a new contribution.
HTTP Request
POST https://backer.com/restapi/v1/funds/<uuid>/contributions
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
frequency | frequency | payment frequency (only one-time is supported at this time) | |
amount | cents | amount in cents | |
activation_date | date | yes if onetime frequency | |
first_day | day_of_month | yes if recurring contribution | |
second_day | day_of_month | yes if semimonthly frequency |
Update Contribution
curl "https://backer.com/restapi/v1/contributions/56bb5278-1df8-46b2-93a0-dfc312bb54a4" \
-X PUT
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{"amount": 50000}'
The above command returns JSON structured like this:
{
"uuid": "56bb5278-1df8-46b2-93a0-dfc312bb54a4",
"frequency": "monthly",
"amount": 50000,
"status": "scheduled",
"activation_date": "2022-10-14",
"first_day": 14,
"second_day": null
}
This endpoint updates an existing contribution.
HTTP Request
PUT https://backer.com/restapi/v1/contributions/<uuid>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of contribution to update |
Query Parameters
Parameter | Type | Required | Description |
---|---|---|---|
frequency | frequency | payment frequency | |
amount | cents | amount in cents | |
activation_date | date | yes if onetime frequency | |
first_day | day_of_month | yes if recurring contribution | |
second_day | day_of_month | yes if semimonthly frequency |
Cancel Contribution
curl "https://backer.com/restapi/v1/contributions/56bb5278-1df8-46b2-93a0-dfc312bb54a4" \
-X DELETE
-H 'Content-Type: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
The above command returns JSON structured like this:
{
"uuid": "56bb5278-1df8-46b2-93a0-dfc312bb54a4",
"frequency": "monthly",
"amount": 50000,
"status": "cancelled",
"activation_date": "2022-10-14",
"first_day": 14,
"second_day": null
}
This endpoint cancels a contribution.
HTTP Request
DELETE https://backer.com/restapi/v1/contributions/<uuid>
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of contribution to cancel |
Create Payment (not supported yet)
curl "https://backer.com/restapi/v1/contributions/56bb5278-1df8-46b2-93a0-dfc312bb54a4/payments" \
-X POST
-H 'Content-Type: application/json'
-H 'Accept: application/json'
-H "Authorization: 109cb6fd-5658-4297-9bbf-8fa42be80f6c"
-d '{
}'
The above command returns JSON structured like this:
{
"uuid": "56bb5278-1df8-46b2-93a0-dfc312bb54a4",
"frequency": "monthly",
"amount": 15000,
"status": "scheduled",
"activation_date": "2022-10-14",
"first_day": 14,
"second_day": null
}
This endpoint creates an initiated payment for the contribution. This endpoint should be used when the partner is responsible for scheduling contribution payments.
HTTP Request
POST https://backer.com/restapi/v1/contributions/<uuid>/payments
URL Parameters
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | yes | UUID of contribution to create a payment for |
Plans
Get Supported 529 Plans
curl "https://backer.com/restapi/v1/plans" \
-H 'Content-Type: application/json'
-H 'Accept: application/json' %>"
The above command returns JSON structured like this:
[
{
"id": 1011,
"name": "ScholarShare College Savings Plan",
"display_name": "ScholarShare College Savings Plan - California",
"state": "CA",
}
]
This endpoint returns the supported 529 plans.
HTTP Request
GET https://backer.com/restapi/v1/plans
Schemas
User Schema
Parameter | Type | Required |
---|---|---|
uuid | UUID | N/A |
inserted_at | datetime (iso8601) | N/A |
external_id | string | yes |
yes | ||
first_name | string | yes |
last_name | string | yes |
middle_name | string | no |
photo_url | URL | no |
Fund Schema
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | N/A | UUID of the fund |
establishment_url | URL | N/A | 529 account identity verification URL for the fund |
established_at | datetime (iso8601) | N/A | date and time when the 529 fund was established (or linked) |
plan_id | integer | no | ID of the 529 plan provider |
plan_provider | string | N/A | provider of the 529 plan |
account_number | string | N/A | 529 account number |
portfolio_label | string | N/A | 529 portfolio |
public_url | string | N/A | URL of the public fund to share with gifters |
is_established | boolean | N/A | was the fund successfully established? |
settled_balance | integer | N/A | current balance in cents |
pending_balance | integer | N/A | current balance and upcoming contributions & gifts in cents |
fund_name | string | yes | name of the fund |
handle | string | yes | unique handle of the fund for the public fund URL |
photo_url | URL | no | photo of the fund beneficiary |
description | string | no | description of the fund displayed on publid fund page |
Fund Establishment Schema
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | N/A | UUID of the fund |
establishment_url | URL | N/A | 529 account identity verification URL for the fund |
fund_name | string | yes | name of the fund |
handle | string | yes | unique handle of the fund for the public fund URL |
Fund Link Schema
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | N/A | UUID of the fund |
fund_name | string | yes | name of the fund |
handle | string | yes | unique handle of the fund for the public fund URL |
plan_id | integer | no | ID of the 529 plan provider |
plan_provider | string | N/A | provider of the 529 plan |
account_number | string | N/A | 529 account number |
Withdrawal Request Schema
Parameter | Type | Required | Description |
---|---|---|---|
uuid | UUID | N/A | UUID of the withdrawal request |
amount | cents | yes | Amount requested to withdraw |
expense_type | expense_type | yes | Type of expense |
Fund Transaction Schema
Parameter | Type | Description |
---|---|---|
uuid | UUID | N/A |
amount | cents | amount in cents |
description | string | transaction description |
occurred_on | date (iso8601) | date the transaction occurred on |
status | transaction_status | status of the transaction |
type | transaction_type | contribution or gift |
frequency | frequency | payment frequency |
Contribution Schema
Parameter | Type | Description |
---|---|---|
uuid | UUID | N/A |
frequency | frequency | payment frequency |
amount | cents | amount in cents |
activation_date | date | date of the next payment |
first_day | day_of_month | day of the month for a recurring payment (monthly, semimonthly, annually) |
second_day | day_of_month | day of the month for a recurring payment (semimonthly only) |
status | contribution_status | status of the contribution |
Plan Schema
Parameter | Type | Description |
---|---|---|
id | integer | ID of the plan |
name | string | Name of the plan |
display_name | string | Display name of the plan |
state | string | State of the plan |
Errors
Example of error response body:
{
"errors": [
"fund_name is required",
"risk_level is required"
]
}
When calling a Backer API endpoint, if an error occurres the response is a JSON object:
Parameter | Type | Description |
---|---|---|
errors | list of strings | list of error messages |
The Backer API uses the following HTTP error codes:
Error Code | Meaning |
---|---|
400 | Bad Request -- Your request is invalid. |
401 | Unauthorized -- Your API token is wrong. |
403 | Forbidden -- You are not allowed to access that data. |
404 | Not Found -- The specified resource could not be found. |
406 | Not Acceptable -- You requested a format that isn't json. |
422 | Unprocessable Entity -- Your request is valid but the operation failed. |
429 | Too Many Requests -- You are exceeding your request rate limit. |
500 | Internal Server Error -- We had a problem with our server. Try again later. |
503 | Service Unavailable -- We're temporarily offline for maintenance. Please try again later. |
Webhooks
Webhooks allow you to receive notifications when certain events happen. This is particularly useful when you want to trigger an action as soon as something happens in Backer without having to poll the API for changes.
You provide your webhook endpoint URL to Backer along with the events to listen to. From then on each of these events are triggered, Backer will send a POST request to your webhook endpoint URL with the event data.
Backer currently supports the following events:
FundEstablished
The user verified their identity from the verification URL and their fund was successfully established.
Parameter | Type | Description |
---|---|---|
uuid | UUID | UUID of the fund |
established_at | datetime (iso8601) | date and time when the 529 fund was established |
plan_provider | string | provider of the 529 plan |
account_number | string | 529 account number |
portfolio_label | string | 529 portfolio |
is_established | boolean | was the fund successfully established? |
fund_name | string | name of the fund |
EstablishFundFailed
The user failed at verifying their identity.
Parameter | Type | Description |
---|---|---|
uuid | UUID | UUID of the fund |
is_established | boolean | was the fund successfully established? |
fund_name | string | name of the fund |
PaymentSettled
A payment was just settled, the funds are now on the user's 529 account.
Parameter | Type | Description |
---|---|---|
amount | cents | amount in cents |
description | string | transaction description |
occurred_on | date (iso8601) | date the transaction occurred on |
status | transaction_status | status of the transaction |
type | transaction_type | contribution or gift |
frequency | frequency | payment frequency |